PHPUnit is a widely used testing framework for PHP designed to facilitate unit testing by providing a structured and efficient way to write and run tests. It helps developers ensure that their code is reliable and functions as expected. PHPUnit offers various features, such as test-driven development support, code coverage analysis, and integration with continuous integration systems, making it an essential tool for maintaining high-quality PHP applications.
To install PHPUnit on Ubuntu 24.04, 22.04, or 20.04 using the command-line terminal, you will utilize Composer, the dependency manager for PHP. This method ensures you have the latest version of PHPUnit and its dependencies, streamlining the setup process for effective unit testing.
Install PHPUnit on Ubuntu via Composer
Installing Composer Globally
Begin by downloading the latest version of Composer using the command below. This action fetches the Composer installer script and executes it using PHP:
curl -sS https://getcomposer.org/installer | php
If you encounter any issues, ensure that curl is installed on your system by running:
sudo apt install curl
Once you have downloaded Composer, move the composer.phar
file to a directory within your system’s PATH to run Composer from any location in your terminal:
sudo mv composer.phar /usr/local/bin/composer
This command transfers the composer.phar
file to the /usr/local/bin
directory and renames it to composer
, making it executable globally.
To confirm that Composer is installed correctly, execute the following command:
composer --version
Installing PHPUnit with Composer
After setting up Composer, you can install PHPUnit globally on your system using the command:
composer global require phpunit/phpunit
Executing this command installs PHPUnit, which allows you to leverage its functionalities to enhance your PHP project’s testing regime.
Example output when installing:
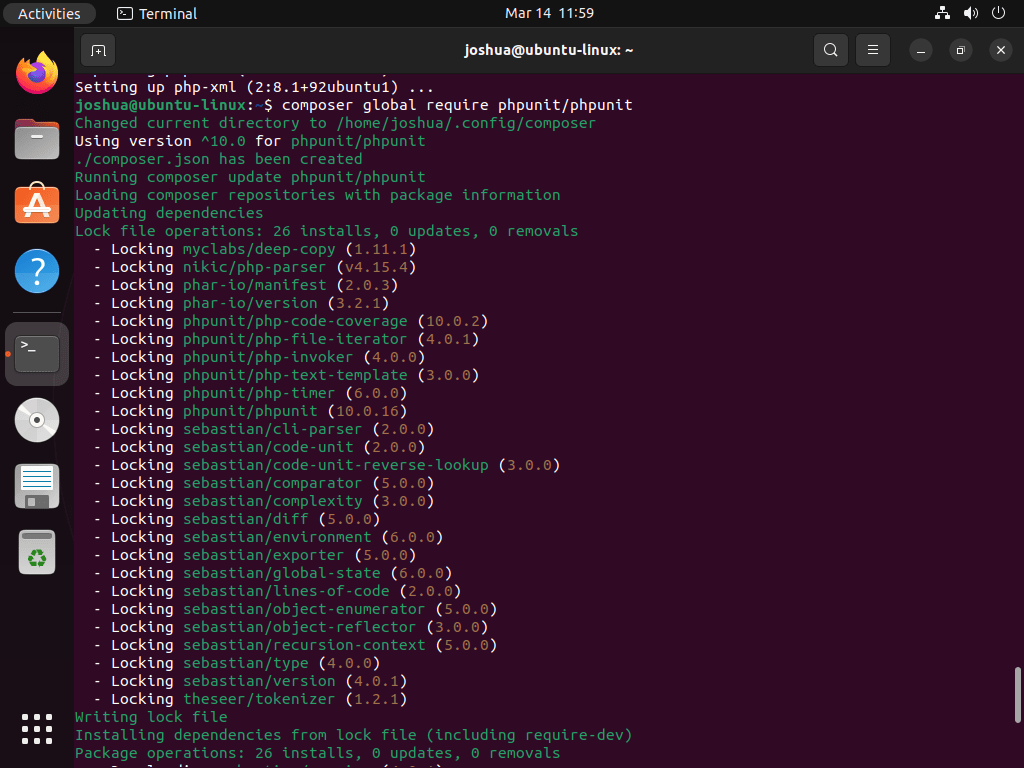
Add Composer Global Bin Directory to PATH
Configuring the PATH Environment Variable
To utilize PHPUnit globally on your Ubuntu system, you must include the Composer global bin directory in your PATH environment variable. This adjustment permits the execution of PHPUnit commands from any directory on your server.
Execute the command below in your terminal to append the Composer global bin directory to your PATH:
echo 'export PATH="$PATH:$HOME/.config/composer/vendor/bin"' >> ~/.bashrc
This command effectively adds the Composer global bin directory to the end of your PATH environment variable, ensuring that the terminal recognizes the PHPUnit commands globally.
Verifying the PATH Update
To confirm that the update to your PATH is successful, display the current PATH variable with the command:
echo $PATH
Running this command outputs the contents of your PATH environment variable, now including the path to the Composer global bin directory. This verification step ensures that your system recognizes the updated PATH, facilitating global access to PHPUnit.
Verify PHPUnit Installation
Running a Test Case to Confirm the Installation
Once you’ve installed PHPUnit via Composer, validating its installation by executing a test case is crucial. Start by creating a new PHP file named test.php in your server’s document root. Open this file with your preferred text editor and insert the following PHP code:
<?php
class Test extends PHPUnit\Framework\TestCase
{
public function testAddition()
{
$this->assertEquals(2+2, 4);
}
}
This code defines a simple test class extending PHPUnit’s TestCase class, with a single test method that asserts 2 + 2 equals 4.
After saving test.php, execute the test by running the following command in your terminal:
phpunit test.php
This command instructs PHPUnit to execute the test defined in test.php. If PHPUnit is properly installed, it will process the test file, execute the test case, and display the results, indicating whether the test passed or failed.
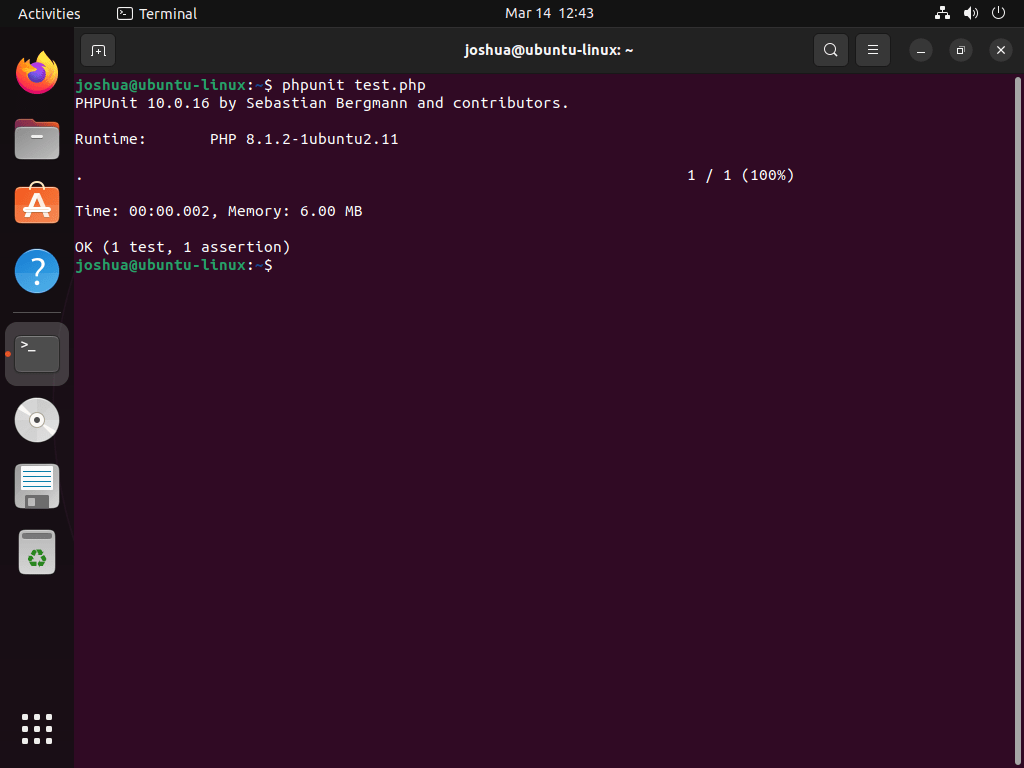
Additional Tips with PHPUnit
Updating PHPUnit
Keeping PHPUnit up to date is essential to leverage the latest features and bug fixes. To update PHPUnit to the most recent version, execute the following command in your terminal:
composer global update phpunit/phpunit
This command instructs Composer to update the PHPUnit package globally to its latest version, ensuring you have access to the most current functionalities and improvements. Regular updates can enhance your PHP projects’ testing efficiency and reliability on Ubuntu.
Removing PHPUnit
If you need to remove PHPUnit from your system, you can do so using Composer. This might be necessary to declutter your development environment or resolve conflicts with other versions. To remove PHPUnit, use the following command:
composer global remove phpunit/phpunit
This command tells Composer to remove the global installation of PHPUnit. After executing this, PHPUnit will no longer be available in your system’s global scope, effectively cleaning up your environment and making room for different testing frameworks or versions if needed.
Conclusion
With PHPUnit successfully installed on your Ubuntu system, you can start writing and running tests to ensure your PHP applications function correctly. Use Composer to manage PHPUnit and keep it updated, taking advantage of its features like code coverage and integration with continuous integration systems. Run your tests regularly to maintain code quality and reliability. For additional help, refer to PHPUnit’s documentation and community resources.